Platform Overview
Fuzzing Terminology
Install the Fuzzbuzz CLI
Find your first C/C++ bug
Find your first Python bug
Find your first Rust bug
Find your first Go bug
Protocol Fuzzing
Seeding your fuzzer
Integrating with libFuzzer
Heartbleed in 5 Minutes
GitHub Integration
GitLab Integration
CLI Integration
fuzzbuzz.yaml reference
Fuzzer Reference
Bug Types
Self-Hosted Fuzzbuzz
Overview
Getting Started
Guides
Tutorials
Integrations
Reference
Find your first bug in Rust
This Getting Started guide will walk you through an end-to-end demo of the Fuzzbuzz platform, from setting up a project all the way to finding and fixing a bug.
Step 1: Get the code
First, clone the tutorial code to your machine:
git clone https://github.com/fuzzbuzz/getting-started-rust
Step 2: Code Review
The repository contains a fuzzbuzz.yaml
file, which is how Fuzzbuzz is configured, along with a couple of Rust files. This section will quickly walk you through what each of these files is used for.
src/broken.rs
This file contains the method we want to test. It contains a very basic bug that will serve the purpose of demonstrating how the Fuzzbuzz platform works.
pub fn broken_function(data: &[u8]) {
if data.len() != 6 {return}
if data[0] != b'q' {return}
if data[1] != b'w' {return}
if data[2] != b'e' {return}
if data[3] != b'r' {return}
if data[4] != b't' {return}
if data[5] != b'y' {return}
panic!("BOOM")
}
fuzz/fuzz_targets/fuzz_broken_function.rs
This file contains fuzz_target
, the fuzz test for broken_function
. The fuzzer will repeatedly run this fuzz target function with new procedurally generated inputs as it attempts to find one that causes a bug.
#![no_main]
use libfuzzer_sys::fuzz_target;
use getting_started_rust::broken_function;
fuzz_target!(|data: &[u8]| {
broken_function(data);
});
fuzzbuzz.yaml
This is the configuration file that tells Fuzzbuzz how to build and test your code. The configuration file below will be sufficient for most Rust projects since Fuzzbuzz automatically detects and runs all cargo-fuzz tests, but you can use the fuzzbuzz.yaml reference if you need more control over your build environment.
tutorial:
language: rust
Step 3: Log in to Fuzzbuzz
Head to https://beta.fuzzbuzz.io and log into your account. If you don't have a Fuzzbuzz account, you can request access here.
Step 4: Upload your code
Fuzzbuzz integrates with your GitHub account to ensure it is always testing your latest changes. The simplest way to test the Getting Started repository on your own account is to fork it.
If you have made changes to the tutorial code, or wish to test your own project instead, just ensure the code is pushed up to a GitHub repository you control, and that it contains a fuzzbuzz.yaml
at its root.
Step 5: Set up your Project
When you first log into Fuzzbuzz, you're presented with a blank homepage and an "Add New Project" button. Click this button, and select "Coverage Guided Fuzzer".

You'll then be presented with a Git Providers screen. Right now Fuzzbuzz supports GitHub, but GitLab and Bitbucket integrations are coming soon.
Select GitHub and press Next.
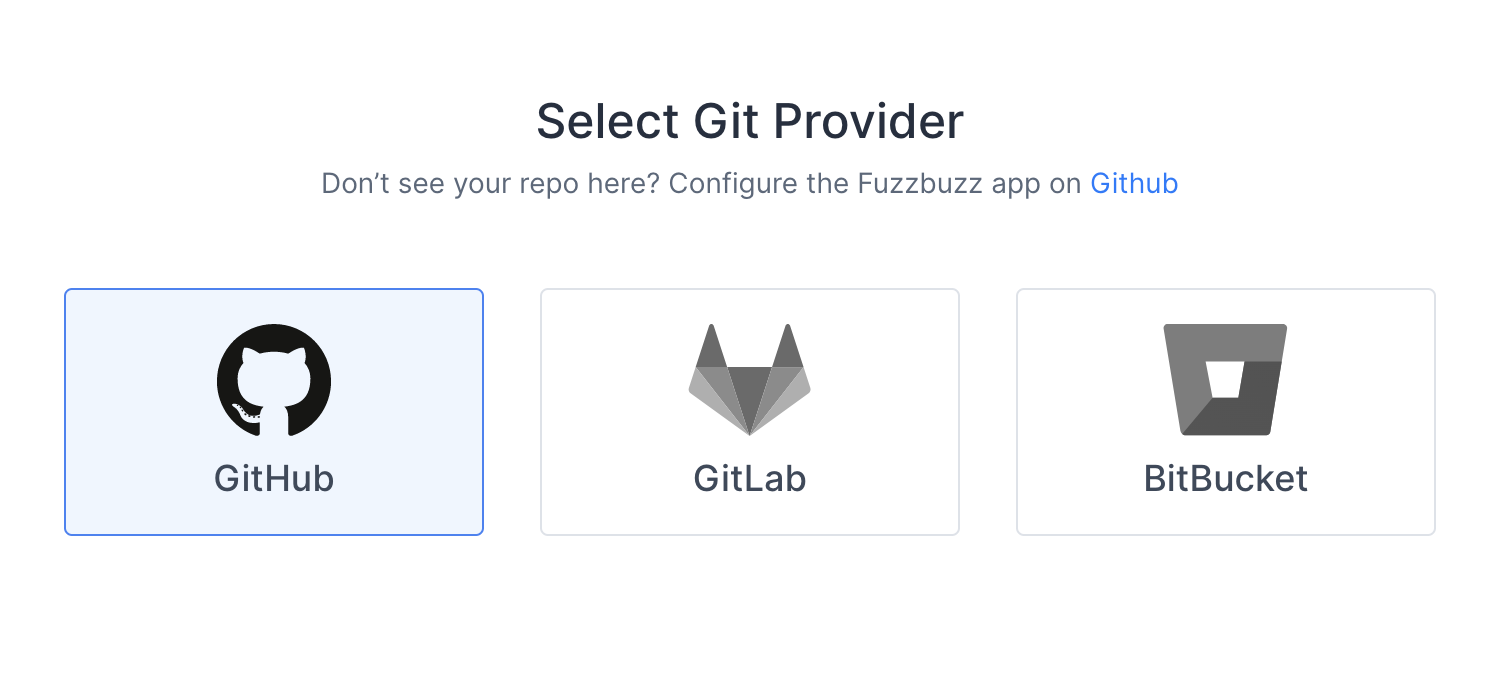
Finally, you will be redirected to GitHub to authorize the Fuzzbuzz app. This initial authorization is used to gather a list of the projects you have access to. You will then be asked to install the GitHub app on your organization or repository.
Once you're finished, select the project you wish to test.
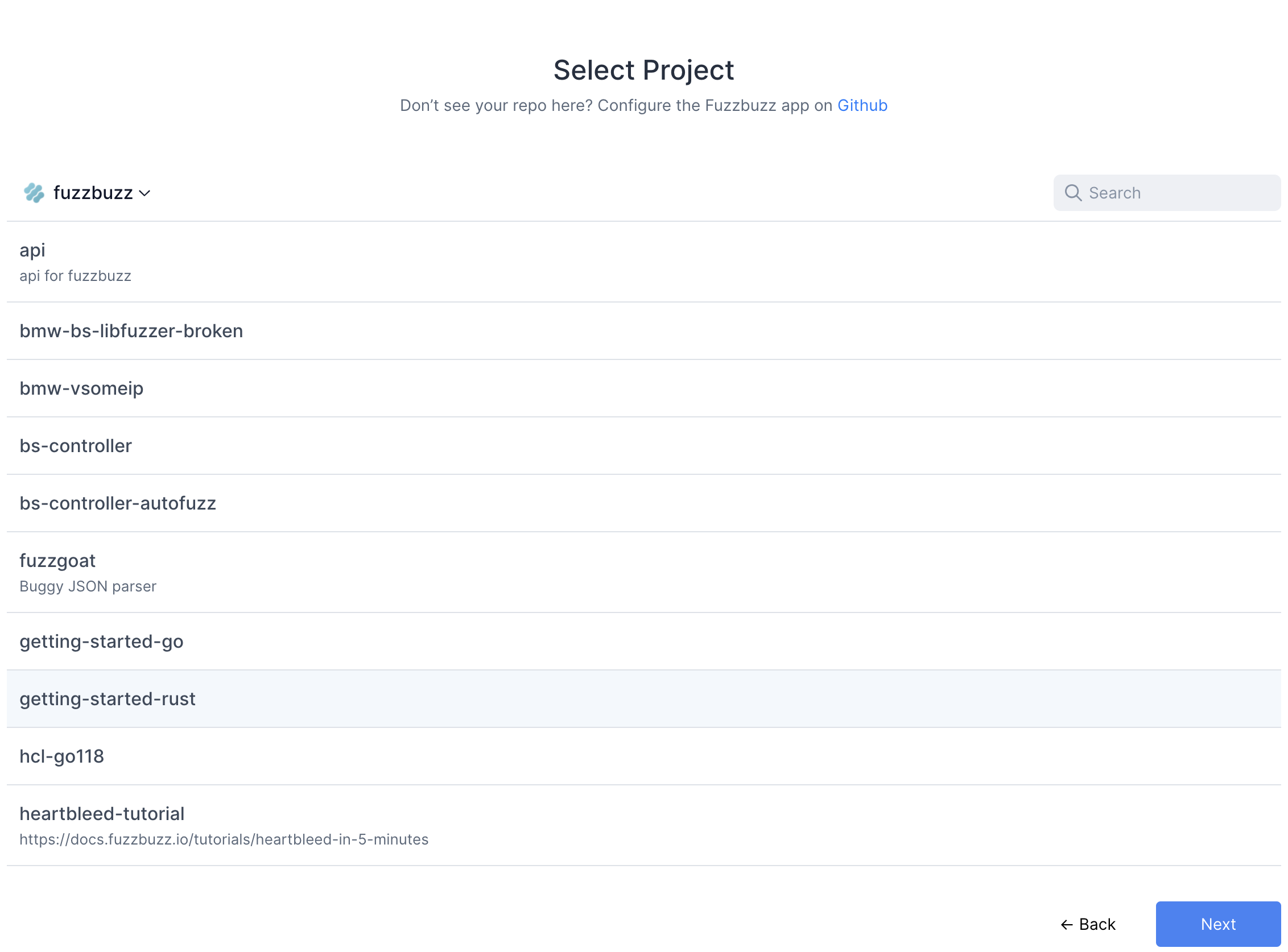
Fuzzbuzz will automatically detect your fuzzbuzz.yaml
file, check it for any errors, and then begin building your code. You can click the job in the Overview page to watch the build logs as your fuzz tests are compiled.
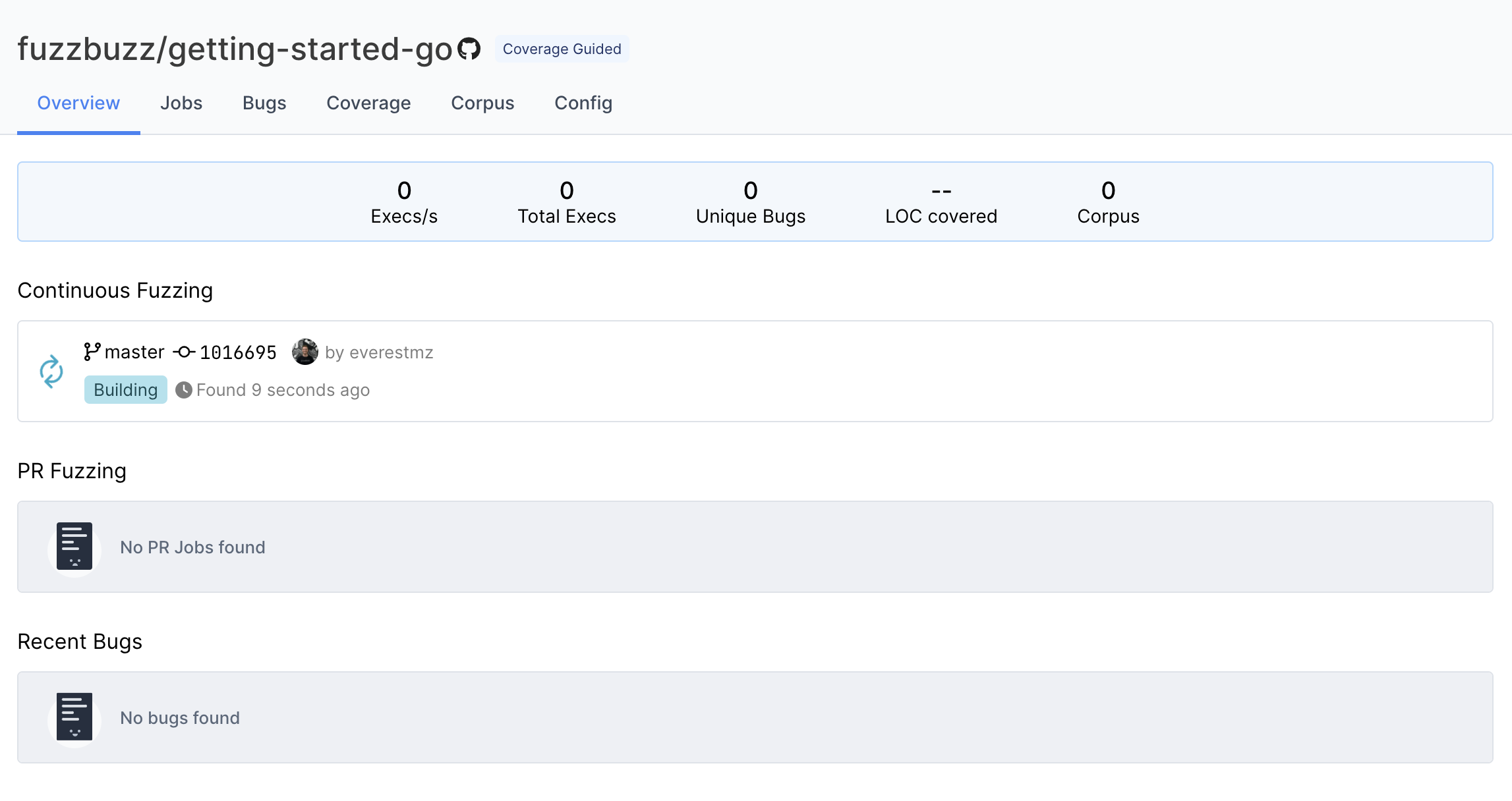
Step 6: Finding bugs
When the build finishes, Fuzzbuzz will automatically detect any fuzz tests in your code, distribute them onto a cluster of CPUs, and begin testing them. Once your fuzz test has started running, you will see some general statistics about the progress the fuzzer is making, and after a few seconds you should see a bug appear. Congratulations - you've just found your first bug with Fuzzbuzz!
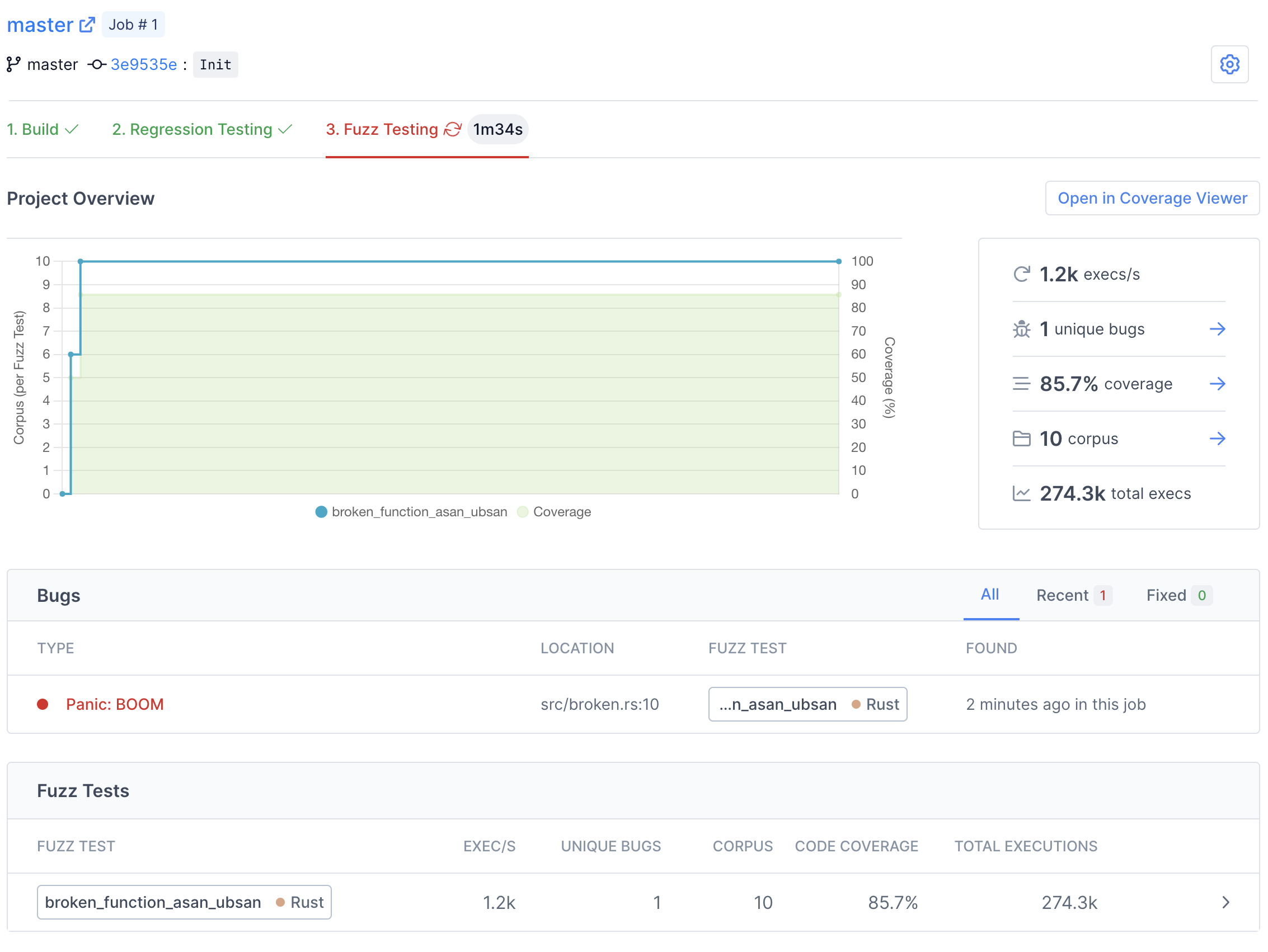
There are many details and features that we'll be skipping over in this tutorial. For more information about the capabilites and features of Fuzzbuzz, head over to the platform overview.
Click on the bug to view more details.
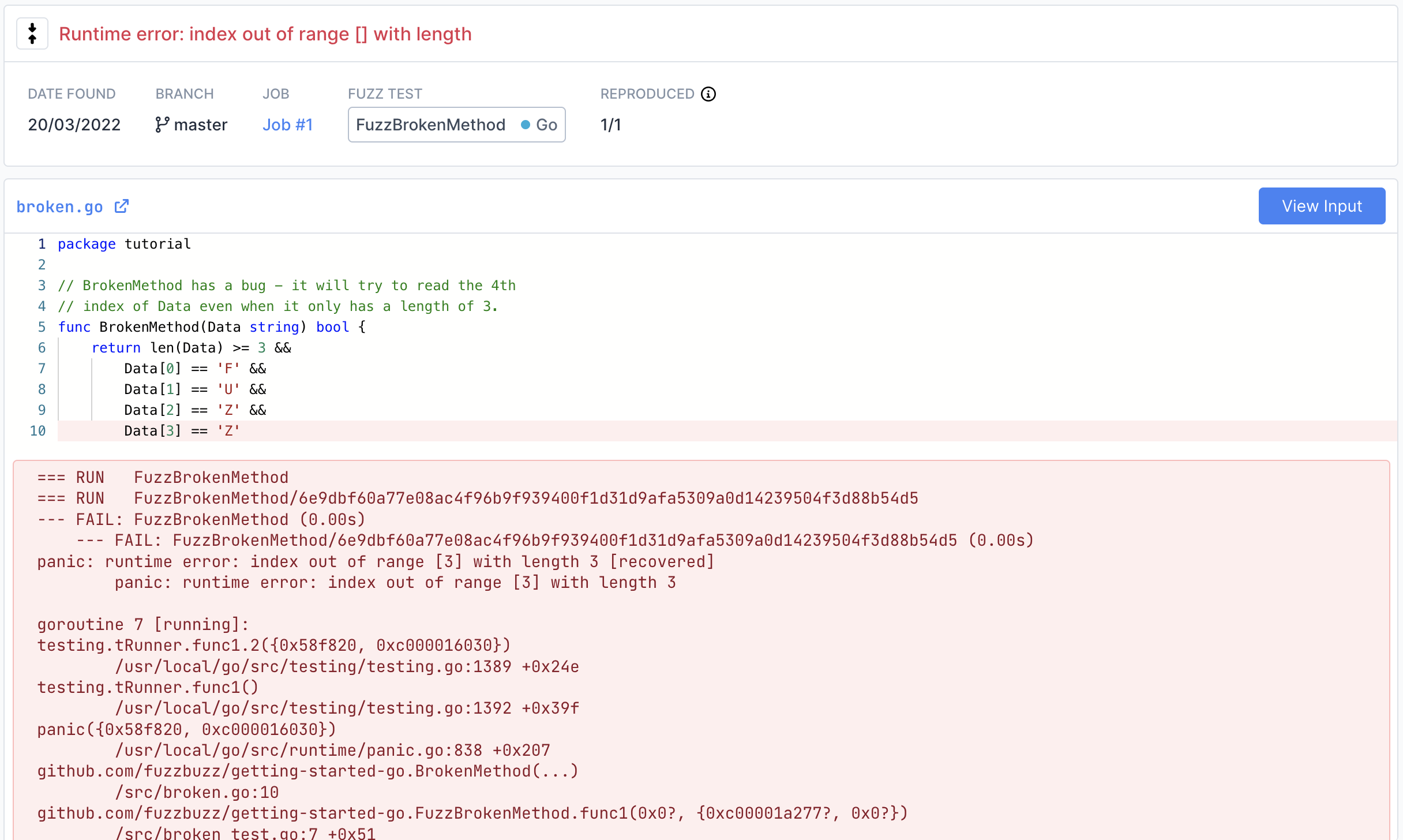
On this page you can find detailed information about the bug, including a stacktrace attached the exact line of code where the bug was encountered.
View the exact input that caused the bug by clicking the "View Input" button in the top right. You will find a Hex viewer you can use to view both the raw and printable forms of the input that caused the bug..
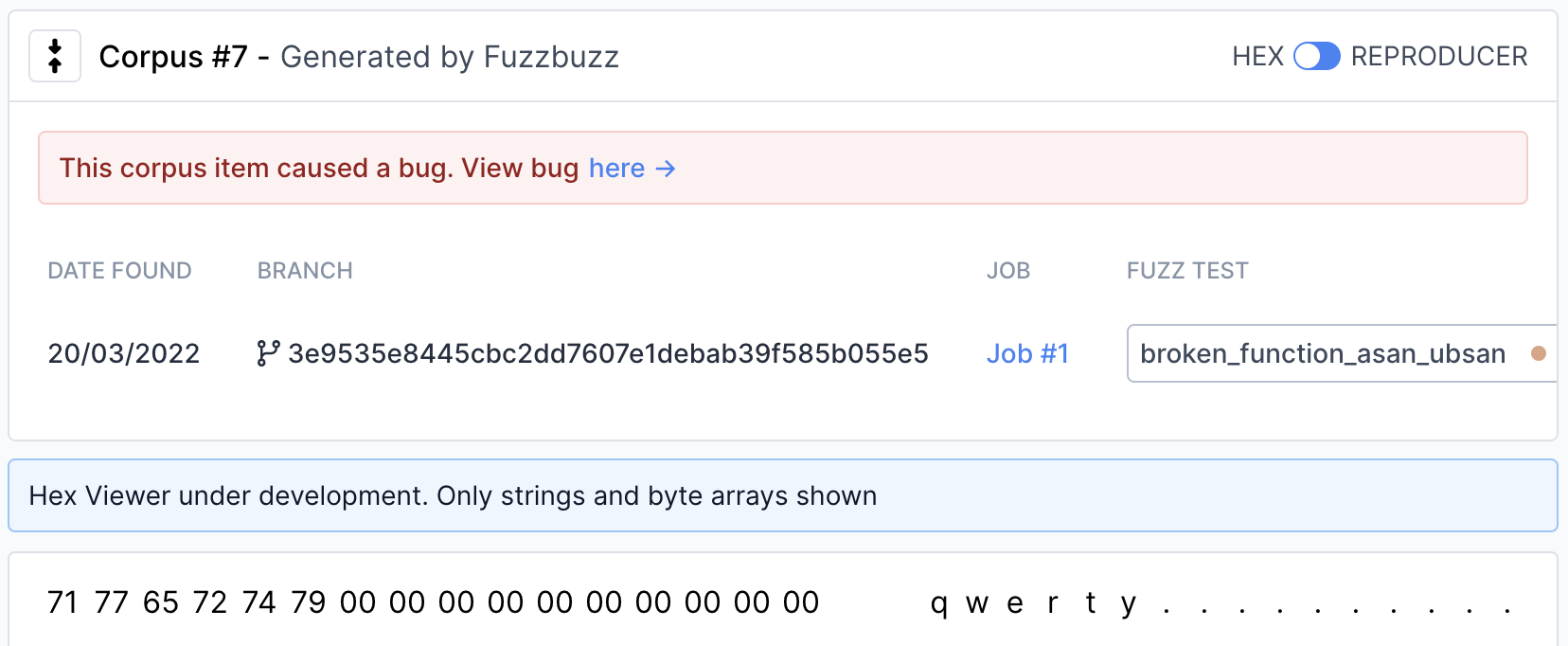
To view instructions on how to reproduce the input locally, move the switch in the top right from "Hex" to "Reproducer".
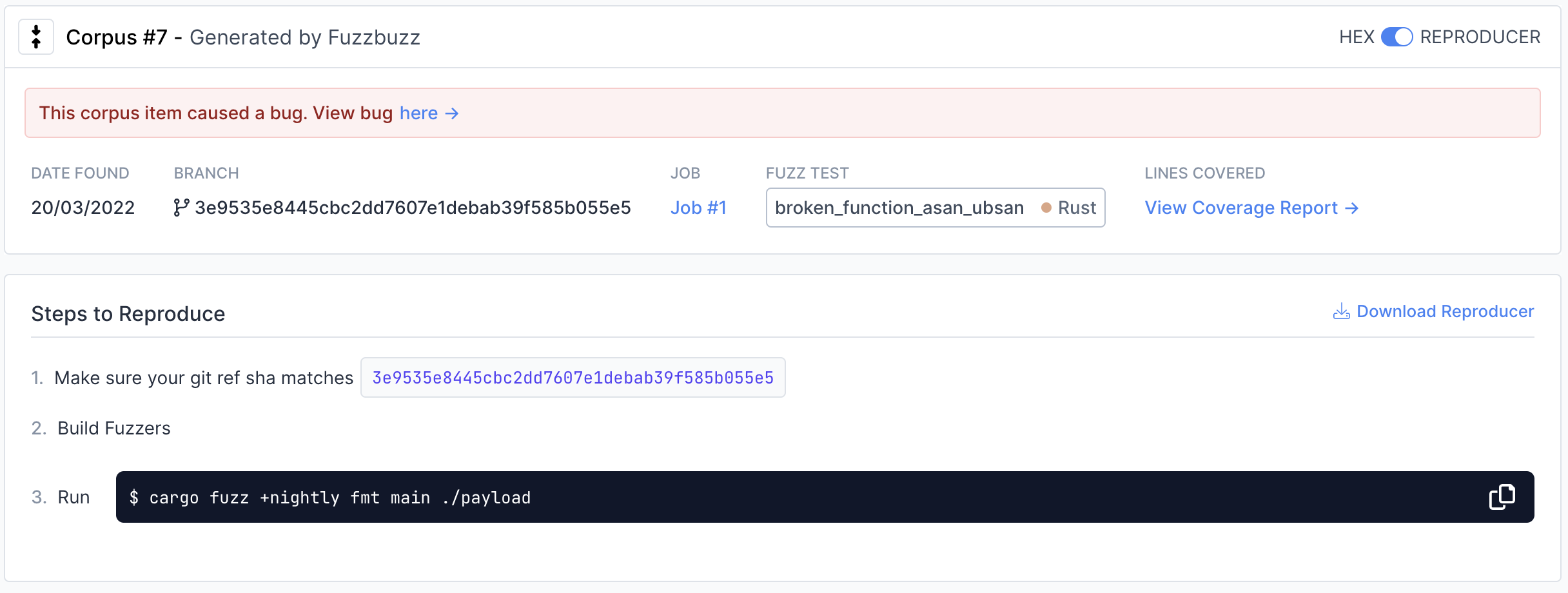
Step 7: Fixing bugs
Now that we've found a bug, it's time to fix it. Open src/broken.rs
, and remove the panic panic!("BOOM")
, so the function looks like this:
pub fn broken_function(data: &[u8]) {
if data.len() != 6 {return}
if data[0] != b'q' {return}
if data[1] != b'w' {return}
if data[2] != b'e' {return}
if data[3] != b'r' {return}
if data[4] != b't' {return}
if data[5] != b'y' {return}
}
Push your code back up to GitHub. Fuzzbuzz will automatically pick up your new change, rebuild your tests, and begin fuzzing the latest version of your code.
After the build finishes, you will notice a new Regression Testing tab.
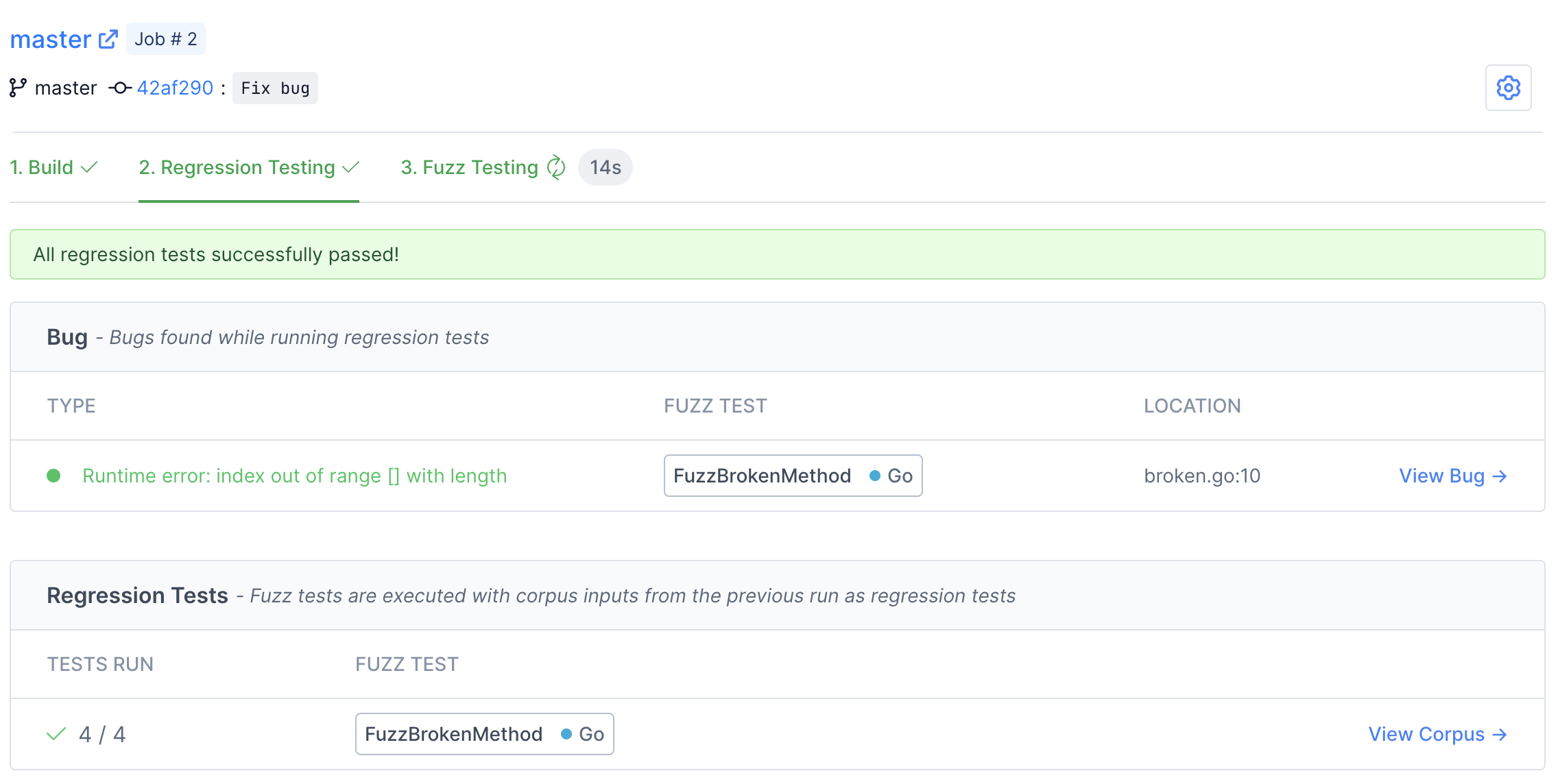
Here you can watch Fuzzbuzz as it runs regression tests on your code. These tests consist of checking if any open bugs have been fixed, old bugs have been reintroduced, or if any previously-found corpus inputs now cause bugs. In this case, you should see the bug we found turn from red to green, indicating that it has been fixed by our most recent change.
Once regression testing has finished, Fuzzbuzz will automatically start fuzz testing your most recent commit.
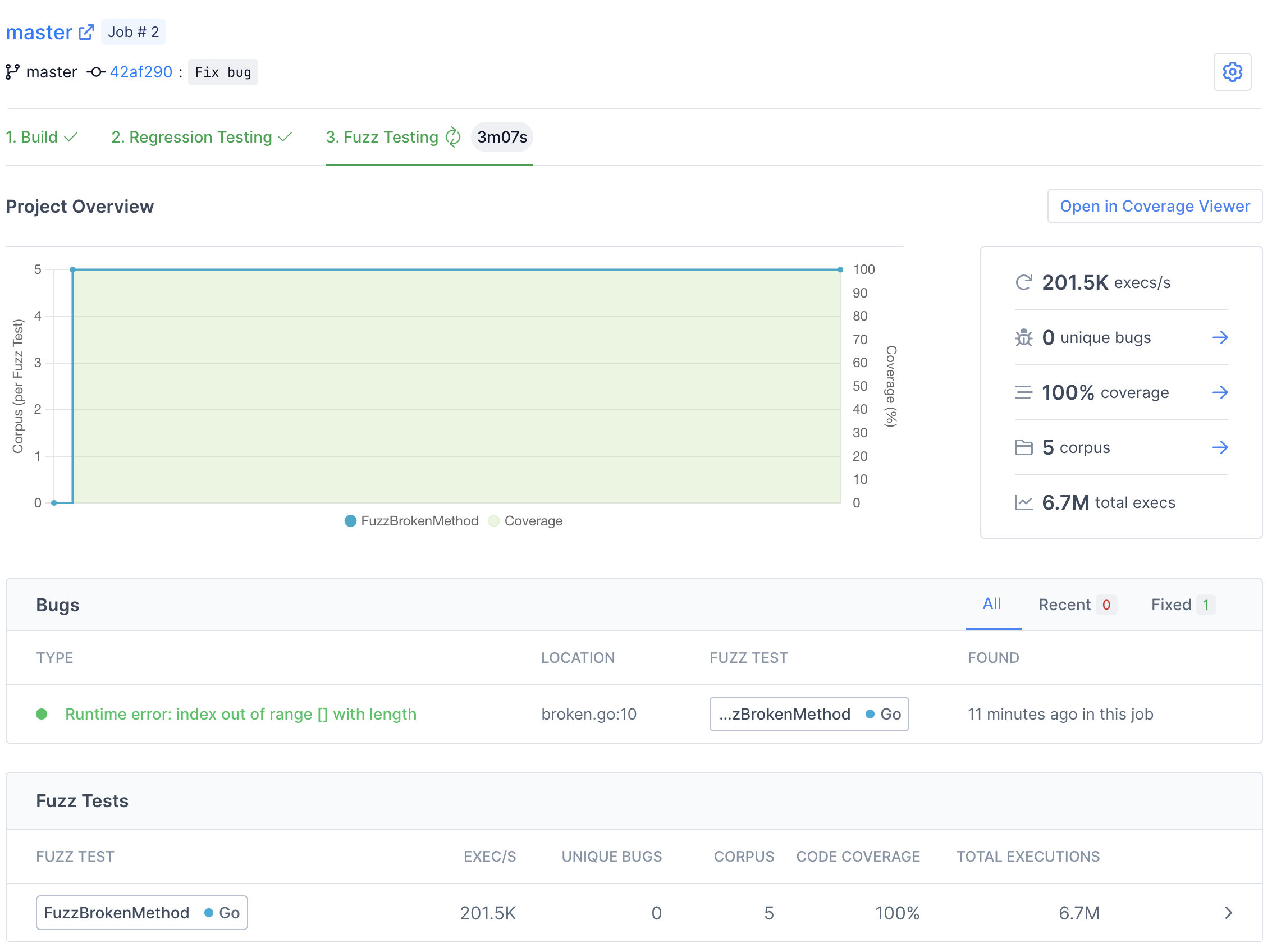
Step 8: Next steps
You've learned the basics of how to set up fuzz tests on Fuzzbuzz! Consider checking out our Platform Overview for a more in-depth look at all the features Fuzzbuzz has to offer, like our code coverage viewer and integrations.